3 Essential Skills To Survive Embedded Career
π Computer Science
π Electrical Engineering
π A third field depending on your industry
Take a look at this interesting diagram:
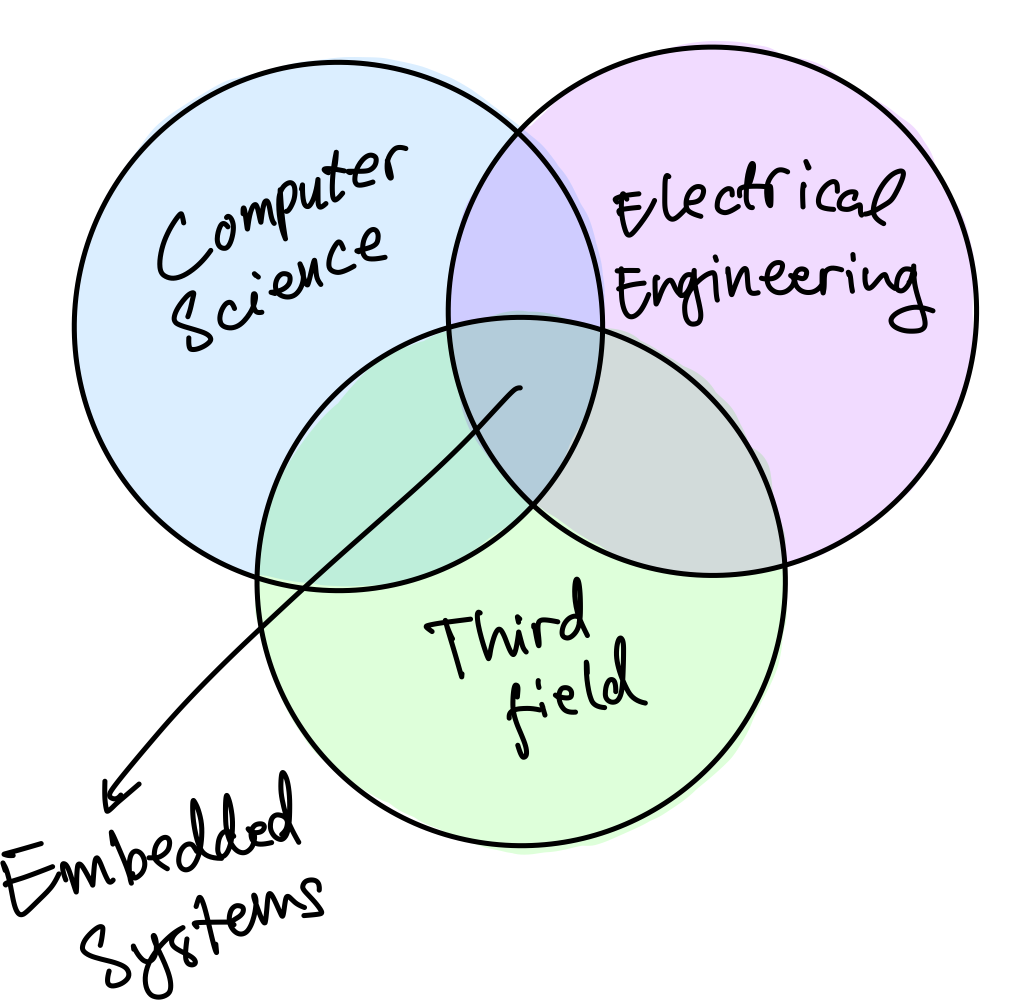
We need EE because we will be interfacing with electronic devices like sensors and actuators.
Understanding the electronics under the hoods will make your life as an embedded engineer easy.
Lastly, we need a third field. It means that if we work on embedded devices on vehicles then we need to learn and understand automotive: its standards, the rules, the principles in auto.
The same with other fields.
I used to work in mining and marine, so I need to learn and understand how mining processes are done, how harsh the environment is, etc.
Now, to help you understand the concept, let's use some examples.
Why CS is Important (Big-O Notation Example)
Here's a simple example you can immediately understand why learning CS is important as an embedded engineer.
Let's say you're writing code for calculating average of temperature data daily. Data comes every second and the type is float. Here's what a junior engineer might write:
float tempAverageC = 0.0;
float tempDataC[1000] = {0.0};
int tempDataCount = 0;
void calculateTemperatureAvg() {
// Fetch the temperature data
float currentTempC = getTemperatureC();
// Insert into the array
tempDataC[tempDataCount] = currentTempC;
tempDataCount = (tempDataCount + 1) % 1000;
// Calculate the average
float tempSum = 0.0;
for (int i = 0; i < tempDataCount; i++) {
tempSum += tempDataC[i];
}
tempAverageC = tempSum / tempDataCount;
}
I already can see 3 major issues:
- The code isn't calculating data average. It's calculating 1000-second window average (which is about 16 minutes). Different result.
- If the buffer is increased to 86400 elements (to match daily/24-hour window), it would be extremely inefficient. Float is usually 4 bytes in 32-bit microcontroller. So, 86400 x 4 = 337.5 kB of memory just to calculate average? Come on.
- If you analyze the time complexity (Big-O notation), you'll get O(n). Which is tolerable in some cases, but not that good for this small function.
You can instantly improve the code by just calculating average without any buffer:

And turn it into code:
float tempAverageC = 0.0;
int tempDataCount = 0;
void calculateTemperatureAvg() {
// Fetch the temperature data
float currentTempC = getTemperatureC();
// Calculate the average
tempAverageC = ((tempAverageC * tempDataCount) + currentTempC) / (tempDataCount + 1);
// Increase counter
tempDataCount = tempDataCount + 1;
}
Β Much better, because it's:
- No longer limited to 1000-second window.
- Only using 8 bytes of storage.
- Time complexity improved from O(n) to O(1) which is constant time.
We can also add resetTemperatureAvg()
to reset the tempAverageC
and tempDataCount
and call it after 24-hour window.
Time complexity isn't commonly discussed in EE major. Usually this is a CS subject. And it's not just limited to time complexity analysis. You have to get good basic CS skills like:
- Space complexity
- Cyclomatic complexity (ever heard of that before?)
- Modular software design
- Interface design
- Static program analysis
- Operating systems concepts
- Program compilation process
- Data structure and algorithms
- etc.
No, I didn't mean you have to be an expert. I said you have to be familiar with CS subjects so you can write much better embedded software.
Why EE is important (Energy Monitoring Example)
Let's say engineer G was asked to build an embedded system to monitor energy consumption.
He had finished the code:
- Beautiful
- Elegant
- Easy to read
- Easy to change
Just perfect. But somehow he couldn't correctly read the current power value in watt.
Btw he used something like this to read the electrical current:
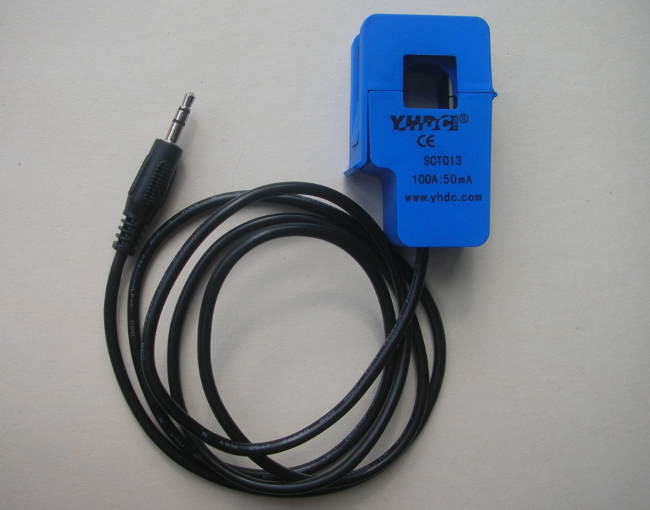
Pretty standard.
Upon inspection, it turned out he had connected the sensor directly to the microcontroller ADC (Analog-to-Digital Converter) pin, and hoping that the signal would be just "there".
Engineer G thought that every ADC could read any signals. He wasn't aware that the signal he wanted to read was a AC signal, not DC. So, to solve his problem, he should understand first the concept of Signal Conditioning.
In EE, we learn that signal should be processed before we can obtain meaningful data. In this example, that means we should understand:
- That the the signal is AC, not DC
- The signal should be read by bi-directional ADC, not built-in ADC
- The ADC should capable of sampling at least 2x the signal frequency (Nyquist theorem), but that's the theoritical minimum. Realistically we need 10x sampling rate
- The signal should be amplified (if necessary), which means designing signal amplifier
- The signal might contain noise, so it's necessary to filter unwanted noises (using analog filter, or Fast-Fourier Transform) and extract just the important part, which is 50Hz or 60Hz signal.Β
Only then we can get valid electrical current data and calculate the power (in watts).
That's why you can't just graduate with CS degree and work immediately as an embedded engineer. You need some EE basics.
Why CS + EE are important (Struggling Engineers Example)
Meet Bob and Arjun.
Bob is a software engineer, he thinks that writing well-crafted software is all he needs, so he learns and practices:
- Clean and beautiful software architecture
- Best design patterns and each use case
- Algorithms for many problems
While his software is beautiful and readable, he is also:
- Struggling to bring up a new development board his boss asked 2 weeks ago
- Confused why the device he is working on cannot last long, even with a 10000mAh battery
- Angry when the sensor is outputting messy data that breaks his algorithm
Bob is good at CS, but bad at EE.
Arjun is good at EE, but bad at CS.
Arjun believes that hardware is everything. So he knows inside out about embedded hardware, analog circuit, etc.
But because his software skill is weak, the firmware he wrote is hard to maintain and unreadable. Arjun needs to consult a lot to Bob about modular firmware design, and coding best practices.
Neither Arjun or Bob is a good embedded systems engineer. But once they learn the complementary skills (EE for Bob and CS for Arjun), they are essentially unstoppable.
That being said, if you're good at EE and CS, you won't survive long without the 3rd Field.
Why EE + CS + 3rd Field are important (IoT Example)
I'll show you why 3rd field is that important.
I'll use IoT as an example. Now, let's dissect IoT into 3 pillars:
- CS: Firmware development for the device.
- EE: Hardware development and sensor design.
- 3rd Field: Data engineering
Yes, IoT is actually a DATA industry. Embedded system in this case is supporting the collection, storage, and transmission of sensor data.
Yes you need knowledge about things like BLE, WiFi, LoRaWAN, ZigBee. But actually those are not IoT-specific knowledge.
If you're building custom IoT device hardware, you need strong technical knowledge about CS, EE, and data simultaneously.
As I said, usually IoT device is responsible for collecting, storing, processing, and transmitting data, which usually comes from the sensors (e.g. temperature, humidity, light, electrical voltage and current, etc). Let's see what data has to do with each stage:
- Collecting: You need to understand data filtering, handle noisy data, and callibration. You can't effectively do this stage if you don't understand data collection principle.
- Storing: What is the efficient way of storing data? What if your data is too big for the device to store? How you secure the stored data? What if someone tries to steal the data? Do you have a small database engine inside the device? Is it okay if some of data lost/missing? How do you store data from 12 different sensors? You need to know basic data engineering.
- Processing: Do you know how to calculate common statistics of the data (average, max, min, mean, standard deviation)? How would you compress big data? Do your device need to visualize data? Can you interpret the data to alter the device's behaviors? Again, data engineering.
- Transmitting: What data to transmit? In what format? Do you follow any standard to transmit data? When is the right time to transmit? What if the receiver can't receive your data? Do you use exponential-backoff? What if 1000 devices are transmitting data at the same time?
You see, without understanding basic data engineering your EECS skills are incomplete.
This is not including the understanding about software stack to receive, transform, visualize, and extract insights from the data. Things like Thingsboard, NodeRED as the dashboard, Grafana as a visualizer, InfluxDB as a time-series database, MQTT Broker to collect data, etc.
Now, replace IoT with your industry:
- Medical
- Automotive
- Aerospace and Defense
- Energy and Utilities
- Agriculture
- Transportation
Get a good grasp of those 3 essential skills, and you'll be truly unstoppable.
Whenever you're ready, there are 2 ways I can help you:
1. Becoming Embedded Freelancer Book. Learn how to earn more money (even more than your main job) working on freelance projects using a proven path explained from an embedded engineer's point-of-view.
2. Firmware Development Workflow Guide. Upgrade your workflow to achieve efficient development, consistent code quality, robust firmware, and skyrocketed your productivity as an embedded engineer.